Rails allow us to update attributes using different instance and class methods, understanding these methods allows us to avoid insane bugs. Let’s explore these methods
attribute=
Syntax:
x = User.first
x.name = 'bhanu' // name= is our attribute=
As you can see this is an instance method and the data is not written to the DB yet. we have to run x.save to save it.
write_attribute
Syntax
x = User.first
x.write_attribute(:name, 'bhanu')
This is also an instance method, and data is not written to DB yet.
attributes= / assign_attributes=
Syntax
x = User.first
x.attributes = { name: 'bhanu', number: '76767667678'}
x.assign_attributes = { name: 'bhanu', number: '76767667678'}
The changes are not written to DB. This method will set the attributes that are passed in the hash, the remaining attributes that we don’t pass will be left unchanged.
update_attribute
Syntax
x = User.first
x.number = '75657565645'
x.update_attribute(:name, 'bhanu')
# Query that will get created is UPDATE "users" SET "name" = 'bhanu', "number": '75657565645', updated_at" = '2021-11-11' WHERE "users"."id" = '1'
This will write the data directly to the DB, it also updates the updated_at field. No Model validation is done and this also updates other attributes which got updated.
In the above example, we intend to update only the name field but as we updated the number field that also got updated. use it with caution
update_columns
Syntax
x = User.first
x.update_columns({name: 'bhanu', mobile_number: '7766775678'})
This executes a direct SQL UPDATE query and bypasses any validations.
update_column
Syntax
x = User.first
x.update_column(:name, 'bhanu')
This behaves the same as update_columns but takes only one field as input.
update_all
This is a class method.
Syntax
User.where(name: "bhanu").update_all(name: "don")
This also executes a direct SQL UPDATE query and bypasses any validations.
update
Syntax
User.update(1, {name: 'bhanu'})
This methods take 2 arguments, the first argument is id and the second is attributes that you want to update. Validation is done and updated_at is updated. A safe method to use.
Cheatsheet
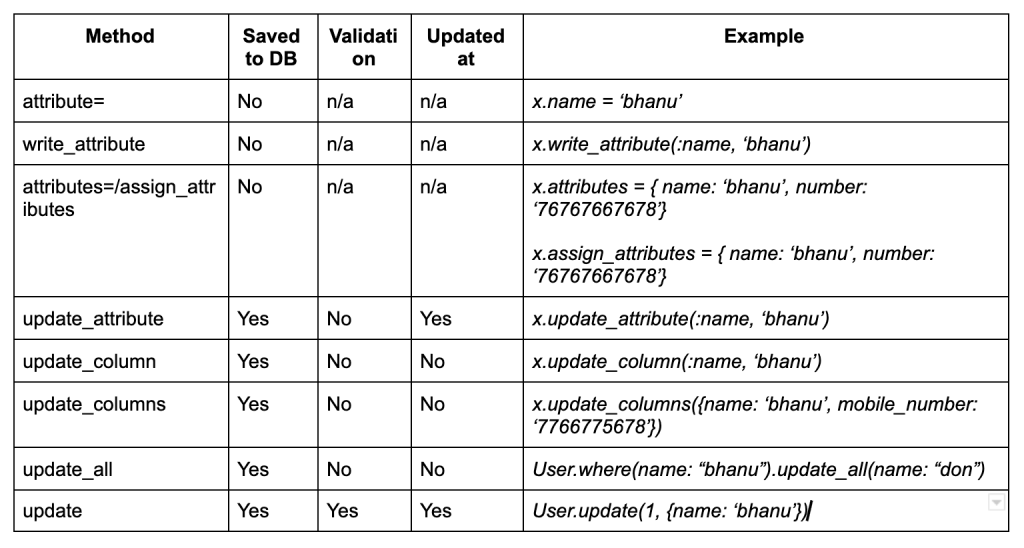