The Challenge
The objective of the minSteps
method is to calculate the minimum number of steps needed to make two strings, s
and t
, anagrams of each other. An anagram is a word or phrase formed by rearranging the letters of another, using all the original letters exactly once.
Breaking Down the Java Solution
public int minSteps(String s, String t) {
int freq[] = new int[26];
char sArray[] = s.toCharArray();
for(int i=0; i<sArray.length; i++) {
int index = sArray[i] - 'a';
freq[index]++;
}
char tArray[] = t.toCharArray();
for(int i=0; i<tArray.length; i++) {
int index = tArray[i] - 'a';
freq[index]--;
}
int count = 0;
for(int i=0; i<freq.length; i++) {
if (freq[i] != 0) {
count += Math.abs(freq[i]);
}
}
return (int)Math.ceil(count * 1.0 / 2);
}
Step-by-Step Exploration
- Frequency Array Initialization:
- An array
freq
of size 26 is initialized to store the frequency of each alphabet. The indexi
corresponds to the alphabet'a' + i
.
- An array
- Counting Frequencies in String
s
:- The characters of string
s
are converted to a character array, and their frequencies are recorded in thefreq
array.
- The characters of string
- Decrementing Frequencies in String
t
:- The characters of string
t
are converted to a character array, and their frequencies in thefreq
array are decremented.
- The characters of string
- Calculating Count of Non-Matching Frequencies:
- The count of non-matching frequencies in the
freq
array is calculated. TheMath.abs
function ensures that both positive and negative differences are considered.
- The count of non-matching frequencies in the
- Calculating Minimum Steps:
- The minimum steps required for anagram transformation are determined by half of the non-matching frequencies. The use of
Math.ceil
ensures that fractional steps are considered.
- The minimum steps required for anagram transformation are determined by half of the non-matching frequencies. The use of
Problem Statement: https://leetcode.com/problems/minimum-number-of-steps-to-make-two-strings-anagram/description/
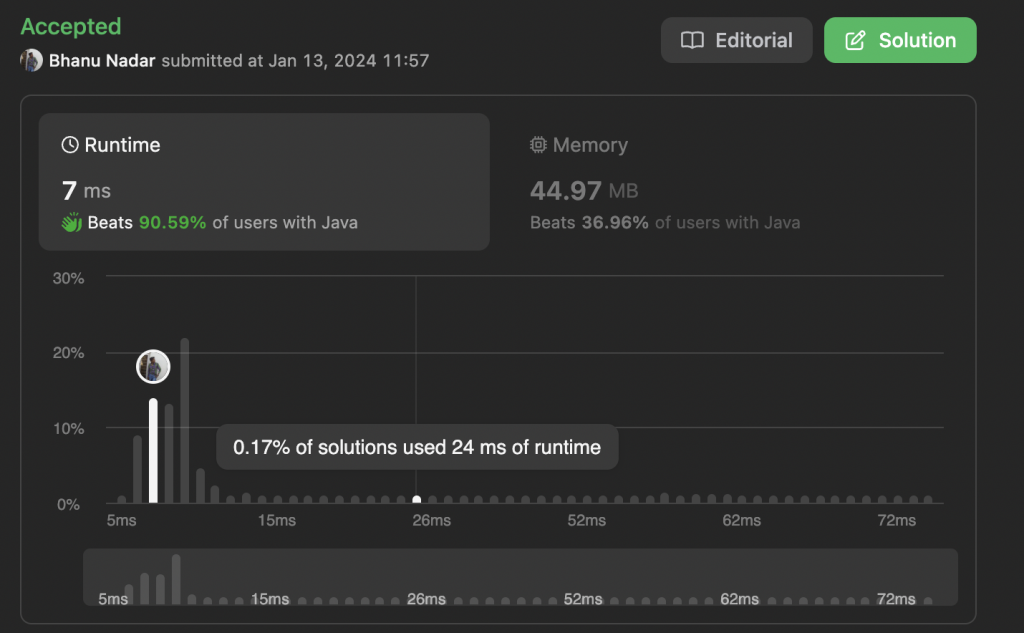
Conclusion
In the realm of anagram minimization, the Java solution showcased in the Solution
class stands as a beacon of efficiency. By cleverly leveraging a frequency array, the algorithm counts the disparities between the strings and determines the minimum steps required. As we traverse the intricacies of algorithmic solutions, this Java gem provides both clarity and effectiveness in tackling the challenge of anagram transformation.