In the dynamic landscape of modern software development, creating applications that are robust, resilient, and user-friendly is paramount. Exception handling stands as a critical pillar in achieving these goals, ensuring that unexpected errors and anomalies are managed gracefully.
we will delve into the intricacies of Micronaut framework, exploring how to effectively handle exceptions at a application level. From understanding the architecture of exception handling to crafting tailored error responses and integrating with logging mechanisms, this blog dissects the key components of Micronaut’s exception handling paradigm.
By the end of this blog, you’ll be equipped with the knowledge to implement a robust global exception handling strategy using Micronaut, ensuring that your applications remain stable, responsive, and user-friendly in the face of unexpected challenges.
Understanding Global Exception Handling:
- A global exception handler is a centralized mechanism that intercepts and manages exceptions that occur during the execution of a program.
- It acts as a safety net, capturing unhandled exceptions and providing a consistent approach to error management throughout the application.
- Having a centralized exception handler allows developers to consolidate exception-handling logic in one location and thus improves readability, maintainability and provides a single point for logging and monitoring exceptions.
- A global exception handler can be customised to generate consistent error responses in a structured format, This ensures that clients receive informative and standardised error messages.
Creating a Global Exception Handler in Micronaut:
- Creating Data Format for Exception Responses
Before diving into the technicalities, let’s establish a consistent data format for error responses. This format encapsulates essential information about the error, such as error code, message, and HTTP status. We define it as a data class using Jackson annotations for serialization. This response can be updated as per your requirement.
import com.fasterxml.jackson.annotation.JsonAutoDetect
import com.fasterxml.jackson.annotation.JsonProperty
import io.micronaut.http.HttpStatus
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.ANY)
data class ErrorResponse(
@JsonProperty("errorCode")
var errorCode: String = "",
@JsonProperty("message")
var errorMessage: String? = "",
@JsonProperty("httpStatus")
var httpStatus: HttpStatus?,
)
2. Creating a Master List for Error Codes
To maintain a systematic approach to error handling, we establish a master list of error codes. While this could be stored in a database or configuration file, for simplicity, we’ll use an enum. This enum holds error code, message, and corresponding HTTP status.
import io.micronaut.http.HttpStatus
enum class MasterErrorCodes(
var code: String,
var message: String,
var httpStatus: HttpStatus
) {
ERR_1000("1000", "BAD Request", HttpStatus.BAD_REQUEST);
fun getMessage(param: String): String {
if (param != null) {
return "$message $param"
}
return message
}
}
3. Creating Custom Exceptions for Domain Logic
Custom exceptions enable us to tailor error handling to specific scenarios. Here, we create a custom exception class that takes a MasterErrorCodes instance and a context parameter. This allows for contextual error reporting.
class CustomException(var error: MasterErrorCodes, var context: String) : RuntimeException()
4. Creating a Custom Exception Handler
Now, let’s delve into the heart of our global exception handling mechanism. We create a custom exception handler class that implements the ExceptionHandler
interface provided by Micronaut. This class handles mapping exceptions to appropriate error responses.
import io.micronaut.http.HttpRequest
import io.micronaut.http.HttpResponse
import io.micronaut.http.HttpStatus
import io.micronaut.http.annotation.Error
import io.micronaut.http.server.exceptions.ExceptionHandler
import jakarta.inject.Singleton
@Singleton
class CustomExceptionHandler : ExceptionHandler<Exception, HttpResponse<ErrorResponse>> {
@Error(global = true, exception = Exception::class)
override fun handle(request: HttpRequest<*>?, exception: Exception?): HttpResponse<ErrorResponse> {
var errorMessage: ErrorResponse
when (exception) {
is CustomException -> {
errorMessage =
ErrorResponse(
exception.error.code,
exception.error.getMessage(exception.context),
exception.error.httpStatus
)
} else -> {
errorMessage = ErrorResponse(
MasterErrorCodes.ERR_1000.code,
exception?.message,
HttpStatus.INTERNAL_SERVER_ERROR
)
}
}
return getResponse(errorMessage.httpStatus, errorMessage)
}
private fun getResponse(httpStatus: HttpStatus?, errorMessage: ErrorResponse): HttpResponse<ErrorResponse> {
// Implement logic to return the appropriate HTTP response based on httpStatus
}
}
In the CustomExceptionHandler
, we capture and process exceptions, converting them into structured error responses using the predefined data format. By categorizing exceptions and associating them with master error codes, we enable precise error identification and user-friendly messaging.
With this comprehensive exception handling setup, Micronaut equips us to deliver robust and reliable applications that gracefully manage exceptions while enhancing user experiences.
Best Practices and Tips
- Use Meaningful Error Codes: Ensure that your error codes are meaningful and easy to understand. They should convey the nature of the error to developers and aid in troubleshooting.
- Keep Error Messages Clear: Craft error messages that are concise, informative, and user-friendly. Users and developers should be able to understand the issue without confusion.
- Customize Exception Messages: Customize exception messages based on the context. Include relevant information, such as the request path or data, to aid in debugging
- Logging and Monitoring: Integrate logging within the exception handling process. Log exception details along with contextual information for effective debugging. Utilize monitoring tools to track the frequency and types of exceptions occurring in the application
- Utilize Enums for Error Codes: Using enums for error codes centralizes their management, making it easier to add, modify, or retire error codes in the future.
- Follow RESTful Conventions: Design error responses following RESTful conventions. Include relevant HTTP status codes and headers for clear communication with clients.
- Externalize Configuration: if error codes and messages might change frequently or need to be localized, consider externalizing them to configuration files or databases
By following these best practices and tips, you can create a robust and effective global exception handling system within your Micronaut application. This system not only enhances error management but also contributes to the overall reliability and quality of your software.
Reference Project: https://github.com/cw-bhanunadar/Micronaut-playground/tree/main
Examples without Exception Handling.
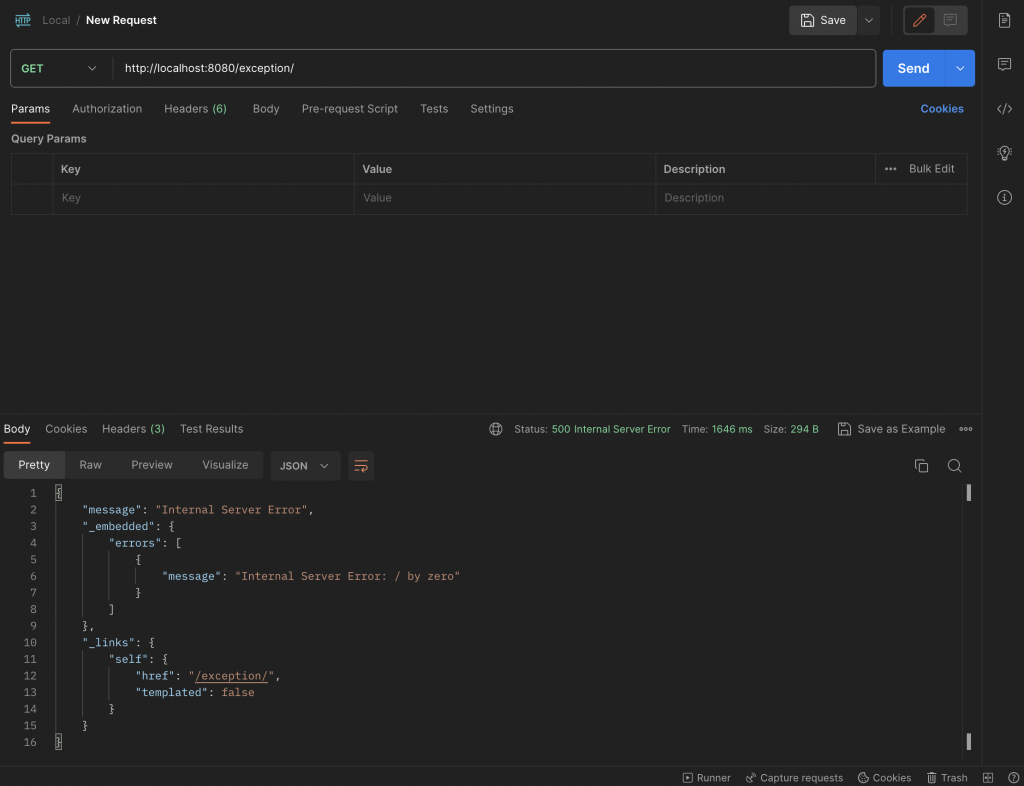
Examples with Exception Handling:
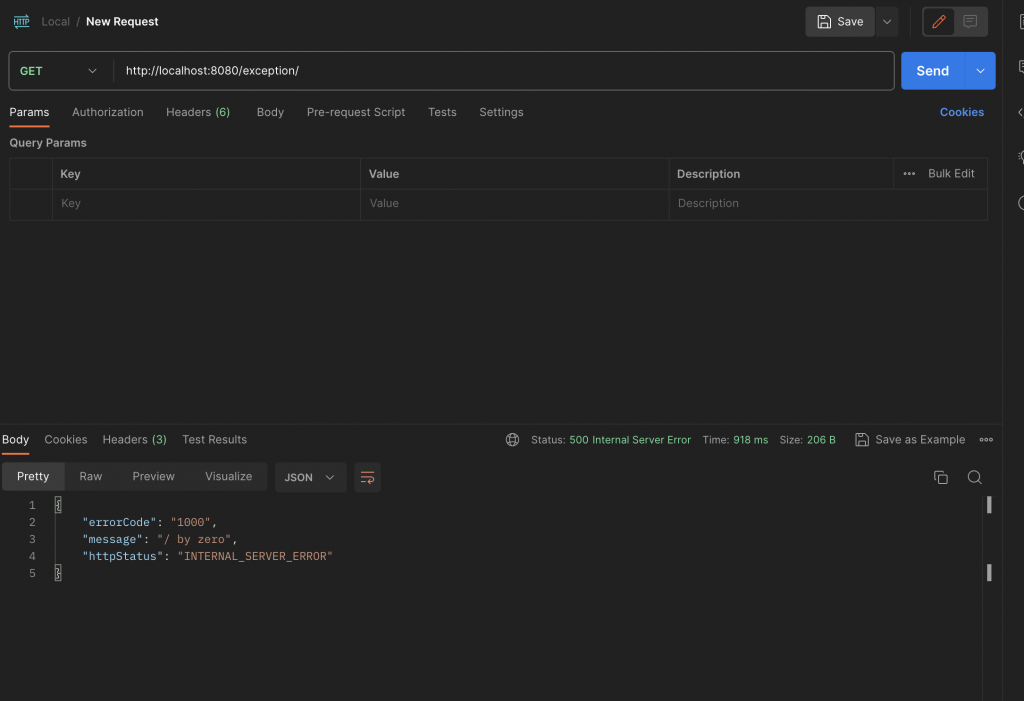
As mentioned, we can use the exception handler in Micronaut to handle all the exceptions happening in HTTP context.