Micronaut, a powerful and lightweight framework, provides an excellent platform for building microservices. In this blog, we’ll explore the seamless integration of MySQL, a popular relational database, into a Micronaut application. This integration ensures data persistence with efficiency and simplicity.
Prerequisites
Before connecting to a database in Micronaut, make sure you have the following prerequisites:
- Micronaut Application: Create a Micronaut project or have an existing one.
- Database: Ensure that you have a running instance of your chosen database system (e.g., MySQL) with a database schema and credentials.
- Docker: Required only if you don’t have MySql instance running.
Steps to Connect to a SQL Database in Micronaut
1. Run MySQL Database
If you already have an instance running you can skip this step. We will use docker to run a MySQL Container on port 3306 with root password as “root”
docker run -d -p 3306:3306 --name mysql-docker-container -e MYSQL_ROOT_PASSWORD=sqlPassword -e MYSQL_DATABASE=my_sql -e MYSQL_USER=sqlUser -e MYSQL_PASSWORD=sqlPassword mysql/mysql-server:lates
2. Add Database Driver Dependencies
Open your project in your favorite IDE, and navigate to the build.gradle
file. Add the MySQL connector dependency to the dependencies
block:
dependencies {
// ...
implementation('io.micronaut.sql:micronaut-jdbc-hikari:4.3.0')
implementation("io.micronaut.data:micronaut-data-jdbc")
runtimeOnly("mysql:mysql-connector-java")
}
3. Configure Database Connection
Micronaut allows you to configure the database connection in the application.yml
(or application.properties
) file. Specify the database URL, username, and password as follows:
datasources:
default:
url: jdbc:mysql://localhost:3306/my_sql?preparedStatementCacheQueries=0
driverClassName: com.mysql.cj.jdbc.Driver
username: sqlUser
password: sqlPassword
dialect: MYSQL
4. Create Model, Controller and Repository
Refer for these details: How to Connect Postgres in Micronaut
5. Check if it’s working
curl --location 'http://localhost:8080/student/' \
--header 'Content-Type: application/json' \
--data '{
"name": "Bhanu"
}'
PR for reference: https://github.com/cw-bhanunadar/Micronaut-playground/pull/17
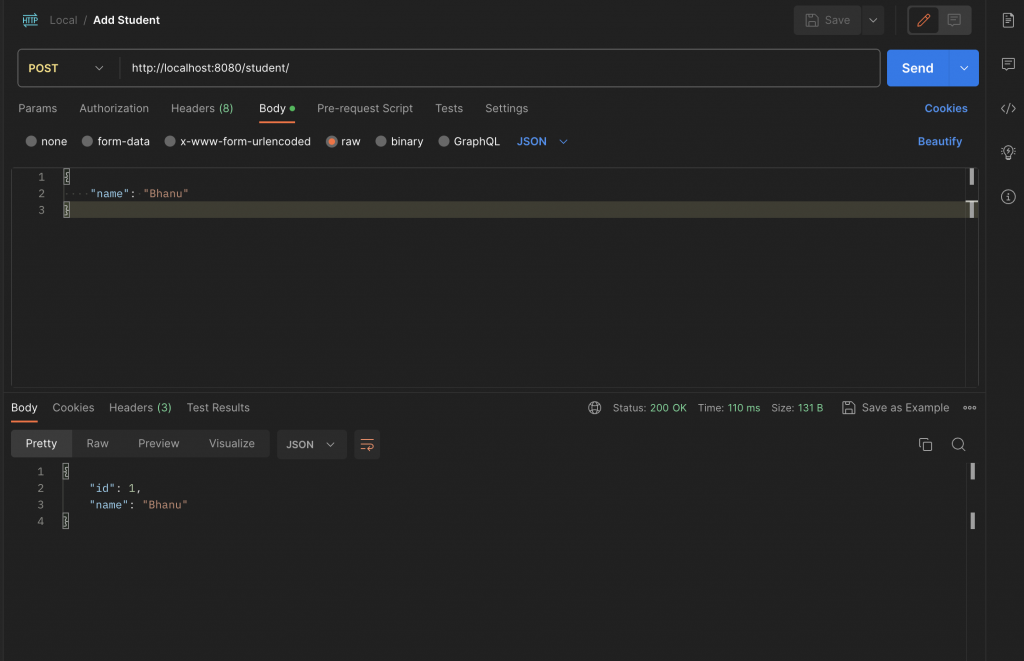