Integrating Sentry with Micronaut allows you to monitor and capture errors and exceptions in your Micronaut applications. Sentry is a popular error tracking platform that helps you discover, triage, and prioritize errors in real-time. Here’s a step-by-step guide on how to integrate Sentry with a Micronaut application
Step 1: Create a Sentry Account
If you don’t have one already, sign up for a Sentry account at Sentry.io.
Step 2: Create a New Project in Sentry
After logging in to your Sentry account, create a new project for your Micronaut application.
Step 3: Get DSN for your SDK
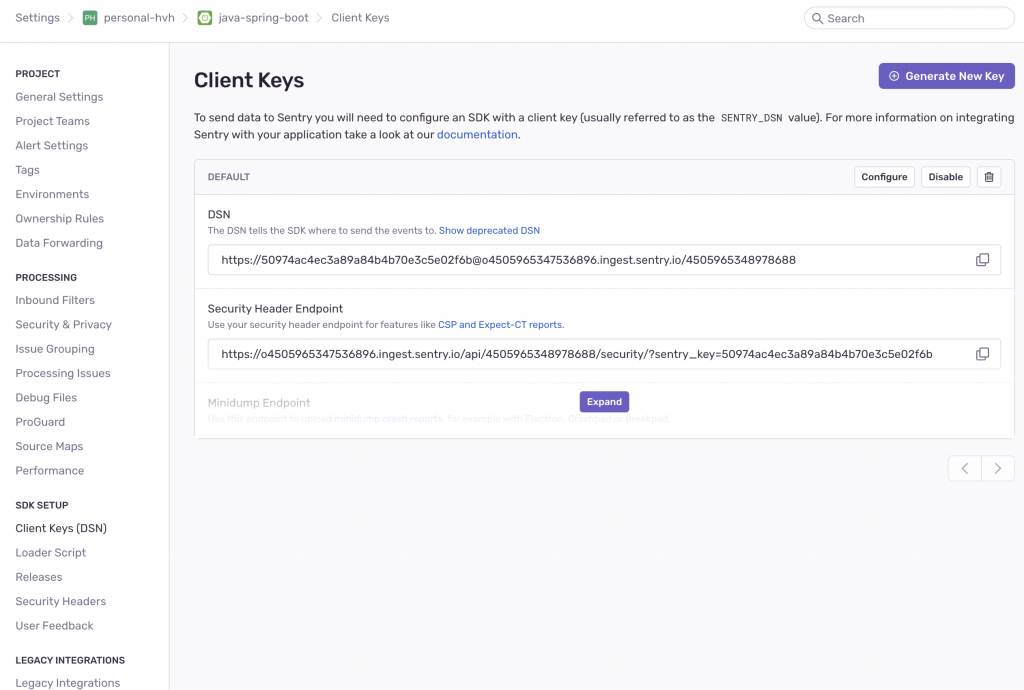
Under Client Keys of your project you will a get a DSN. This DSN is used by the micronaut application to send required information.
Step 3: Add the Sentry SDK as a Dependency
In your Micronaut project, add the Sentry SDK as a dependency. You can do this by adding the following dependency to your build.gradle
(for Gradle) or build.gradle.kts
(for Kotlin DSL):
dependencies {
.... //other dependencies
implementation 'io.sentry:sentry:5.7.4'
}
Step 4: Configure Sentry in application.yml
sentry:
enabled: ${SENTRY_ENABLED:false}
dsn: ${SENTRY_DSN:`https://50974ac4ec3a89a84b4b70e3c5e02f6b@o4505965347536896.ingest.sentry.io/450596534897688`}
Step 5: Create a Sentry Configuration class
package com.example.common
import io.micronaut.context.annotation.ConfigurationProperties
@ConfigurationProperties("sentry")
data class SentryConfig(
var dsn: String? = "dsn"
)
This class will store the data that is defined in application.yml during runtime
Step 6: Initialise Sentry to capture exception
Add Initialisation of Sentry logic during Boostrap of the appilication
package com.example.common
import io.micronaut.runtime.event.annotation.EventListener
import io.micronaut.runtime.server.event.ServerStartupEvent
import io.sentry.Sentry
import io.sentry.SentryOptions
import jakarta.inject.Inject
import jakarta.inject.Singleton
import org.slf4j.Logger
import org.slf4j.LoggerFactory
@Singleton
class Bootstrap {
val log: Logger = LoggerFactory.getLogger("Bootstrap")
@Inject
private lateinit var sentryConfig: SentryConfig
@EventListener
fun onStartupEvent(event: ServerStartupEvent) {
configureSentry()
log.info("This Event got trigger during Startup")
}
private fun configureSentry() {
if (sentryConfig.enabled == true) {
Sentry.init { options: SentryOptions ->
options.dsn = sentryConfig.dsn
// Set tracesSampleRate to 1.0 to capture 100% of transactions for performance monitoring.
// We recommend adjusting this value in production.
options.tracesSampleRate = 1.0
// When first trying Sentry it's good to see what the SDK is doing:
// options.setDebug(true)
}
}
}
}
Step 7: Capture Exception in Exception Handler
Read Also: Mastering Global Exception Handling in Micronaut
You can now use Sentry to capture errors and exceptions in your Micronaut application at a single place using Exception Handler
private fun sendToSentry(exception: Exception?) {
log.error(exception?.message)
Sentry.withScope {
Sentry.captureException(exception!!)
}
}
Code: https://github.com/cw-bhanunadar/Micronaut-playground/pull/4/files
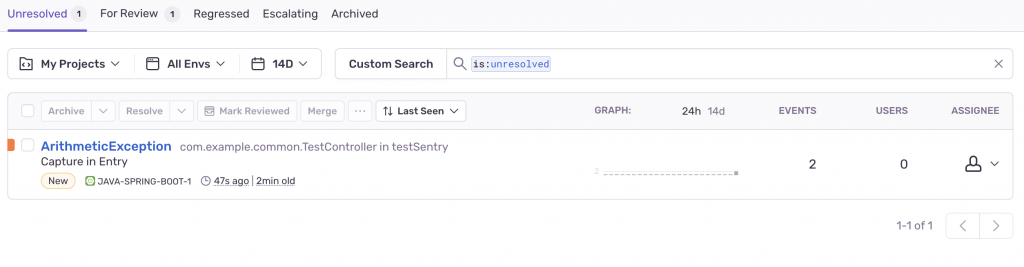